The Ultimate Guide to Converting a Timestamp to Date in Programming Languages
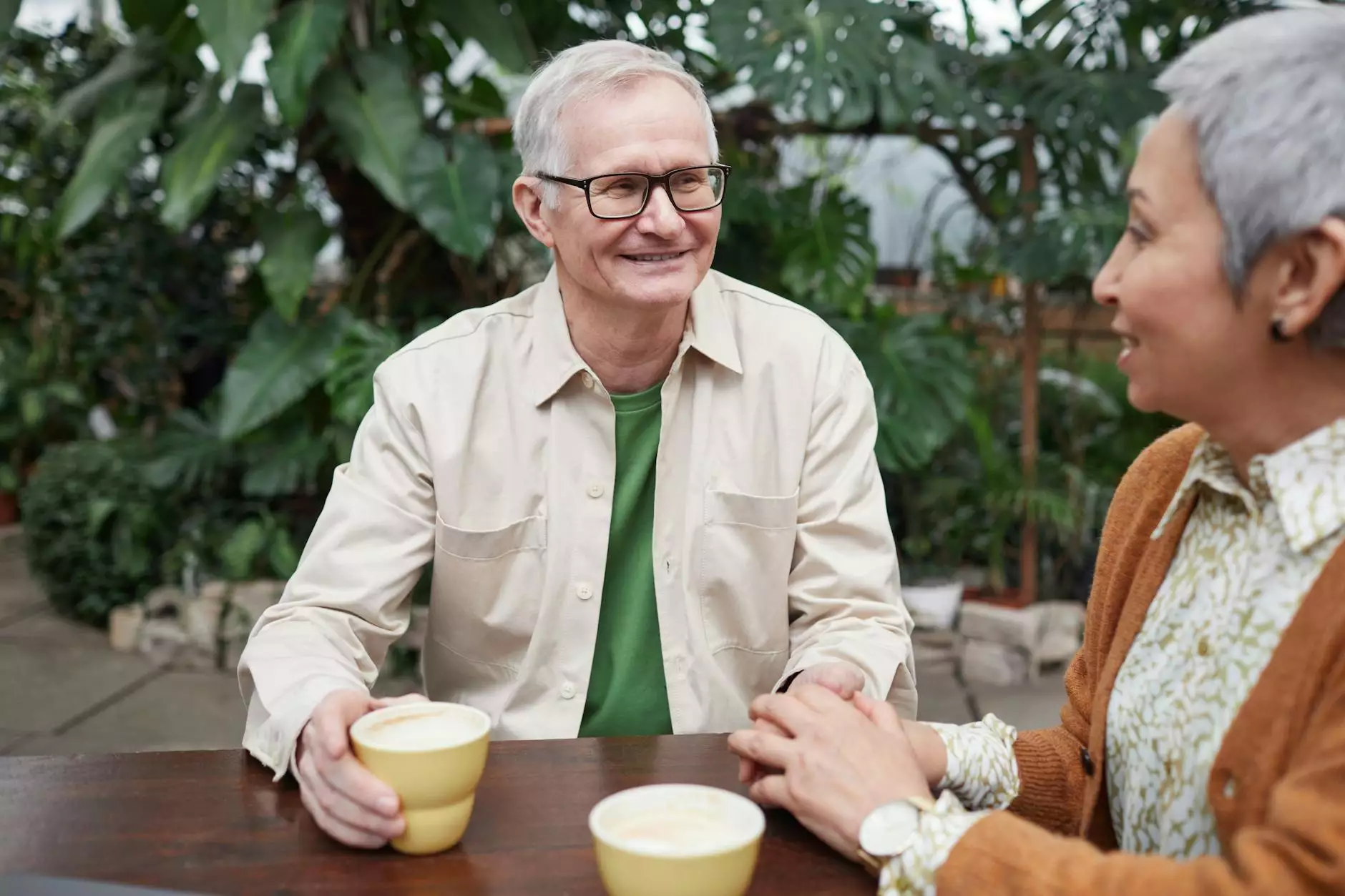
When it comes to dealing with timestamps in programming, converting them to human-readable dates is a common task that programmers frequently encounter. Whether you're working on Web Design or Software Development projects, understanding how to smoothly convert timestamps to dates can significantly streamline your workflow.
Understanding Timestamps and Dates
Before delving into the nitty-gritty details of converting timestamps to dates, let's first establish a clear understanding of what timestamps and dates represent. In programming, a timestamp is typically a numeric value that represents the number of seconds or milliseconds that have elapsed since a specific point in time, often referred to as the epoch. On the other hand, a date is the human-readable representation of a specific point in time, comprising elements such as day, month, year, and time.
Converting Timestamps to Dates in Various Programming Languages
Now, let's explore how you can convert a timestamp to a date in some popular programming languages:
1. JavaScript
JavaScript provides built-in functions like new Date() and toDateString() to facilitate timestamp to date conversions. Here's a simple example:
let timestamp = 1629439200; let date = new Date(timestamp * 1000); document.write(date.toDateString());2. Python
In Python, you can use the datetime module for timestamp to date conversion. Check out this snippet:
import datetime timestamp = 1629439200 date = datetime.datetime.fromtimestamp(timestamp) print(date.strftime('%Y-%m-%d %H:%M:%S'))3. PHP
PHP offers the date function for handling date and time operations. Here's how you can convert a timestamp to a date:
Best Practices for Timestamp to Date Conversion
When working with timestamp to date conversions, it's crucial to keep a few best practices in mind:
- Ensure Proper Timezone Handling: Always consider the timezone implications when converting timestamps to dates to maintain accurate temporal relationships.
- Handle Edge Cases: Be prepared to handle scenarios where timestamps may be null or invalid to prevent runtime errors.
- Optimize Performance: Implement efficient algorithms and libraries for timestamp conversions to enhance the overall performance of your applications.
Enhance Your Programming Skills
By mastering the art of converting timestamps to dates in programming languages, you can elevate your proficiency as a developer and streamline your project workflows. Whether you're engaged in Web Design or Software Development, this knowledge will undoubtedly prove invaluable in your coding endeavors.
Copyright © 2023 SEMalt.Tools. All Rights Reserved.from timestamp to date